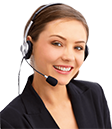
24/7 Customer Support
DumpsMate's team of experts is always available to respond your queries on exam preparation. Get professional answers on any topic of the certification syllabus. Our experts will thoroughly satisfy you.
Site Secure
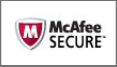
TESTED 01 May 2025